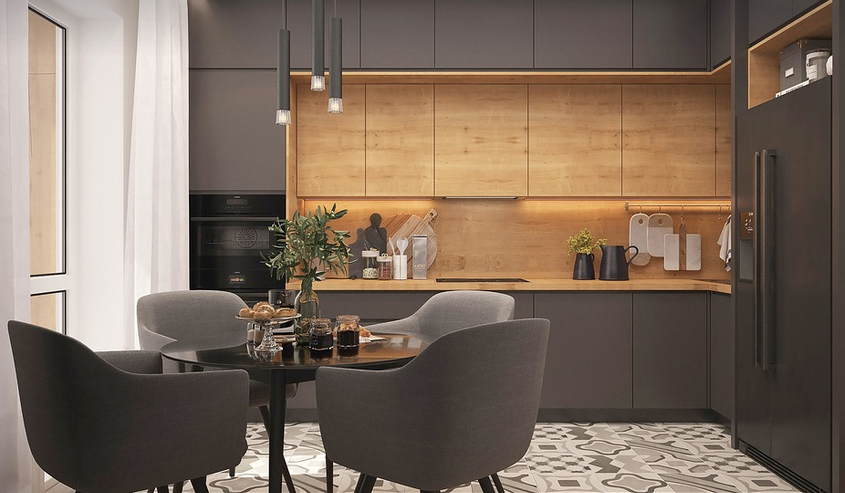
Intro To C++ Programming: Your Guide To Coding Magic
What is C++? A Friendly Introduction
Imagine you want to build something incredible—a website, a video game, maybe even a robot that can cook your breakfast. You have a vision for how it should work, but how do you turn that vision into reality?
That’s where programming comes in. It’s the art of translating those ideas into lines of code, and C++ is one of the most powerful tools in a programmer’s toolbox.
C++ stands for “C plus plus,” and it’s a super versatile language known for its speed, efficiency, and flexibility. Think of it like LEGO bricks—you can combine them to create complex structures or build simple things from scratch.
Why is C++ so powerful? Well, it gives you control over your computer’s hardware and allows for the creation of everything from game-changing software to super-efficient data processing tools.
But enough about theory! Let’s talk about why learning C++ might be just what you need.
**Why learn C++?**
- **Develop Real World Applications:** From mobile apps to complex simulations, C++ powers the future of technology. You can create real things with your coding skills!
- **Become an Efficient Problem Solver:** C++ teaches you how to break down problems into manageable pieces and solve them logically.
- **Gain In-Demand Skills:** The demand for C++ developers is high, making it a valuable skill in today’s job market.
**Where does C++ fit into your life?**
It might seem daunting at first, but C++ opens doors to exciting possibilities. Imagine:
- Building complex games and simulations that push the boundaries of visual realism.
- Designing interactive websites where users can customize their experience.
- Creating innovative applications for mobile devices.
A Quick Tour of C++ Fundamentals
C++ is all about instructions—think of them as the language you use to speak to your computer. But like any language, there are some basics you need to understand before you start creating complex programs.
Let me break down these fundamental concepts to help you get started:
**1. Variables:** Imagine variables as containers that hold pieces of information you want to work with, such as numbers, text, or even images.
- **Data Types:** Think of data types as the labels for your containers; they tell your computer what kind of information is stored in them. You’ll encounter different data types like “int” for whole numbers (like 10), “double” for decimals, and “char” for letters.
**2. Operators:** These are the symbols you use to perform actions on your data, such as addition (+), subtraction (-), multiplication (*), or division (/).
- **Arithmetic Operators:** Plus (+) and Minus (-) for basic math operations.
- **Comparison Operators:** Equal (=), not equal (!= ), greater than (>) , less than (<) to compare values.
**3. Control Flow:** This is about making decisions in your code—like “if this happens, do that” or “if that doesn’t happen, do something else.” You can use control flow statements like “if,” “else if,” and “else” to build complex logic.
- **If Statement:** This lets you execute code only if a condition is met (e.g., if the age of an individual is 18, then let them enter a party).
- **Else If Statement:** This allows you to check for different conditions (e.g., “if the user enters 20 years, then they can access the website.”)
**4. Loops:** Loops are used to repeat actions multiple times, like running a program several times or calculating something many times.
- **For Loop:** This is perfect for iterating a set of numbers (e.g., “for each number in the range 1 to 10, print it”).
- **While Loop:** This allows you to repeat something until a specific condition is met.
**5. Functions and Classes:** These are essential structures for organizing your code and making it more reusable. A function is like a mini-program that performs a specific task, while classes act as blueprints for creating objects that contain data and behavior.
These are just the fundamentals! But don’t worry—you don’t need to master them all at once. Just focus on understanding these core concepts, and you’ll be well on your way to building amazing things with C++!
Get Started: Your First Steps in C++
Ready for your first foray into the world of C++? Here are some simple steps to get you started.
1. **Choose an IDE:** An IDE (Integrated Development Environment) helps with writing, debugging, and compiling code. Popular choices include Visual Studio, Xcode, and Code::Blocks.
2. **Learn the basics:** Explore tutorials, online courses, and resources designed to help beginners grasp the fundamentals of C++. There are plenty of free options available on websites like YouTube, Udemy, and Coursera.
3. **Start Small!** Don’t feel overwhelmed by complex projects at first. Begin with simple programs like “Hello World!”, which just prints a message to the screen.
4. **Practice regularly:** The best way to learn anything is through hands-on experience. Write small programs, experiment, and don’t be afraid to make mistakes (they’re learning opportunities!).
5. **Join the Community:** Connect with other learners! Join online forums or communities specific to C++, seek advice from experienced coders, and share your progress.
Remember: Learning a programming language takes time, patience, and dedication. Don’t get discouraged if you encounter challenges along the way—that’s just part of the learning process.