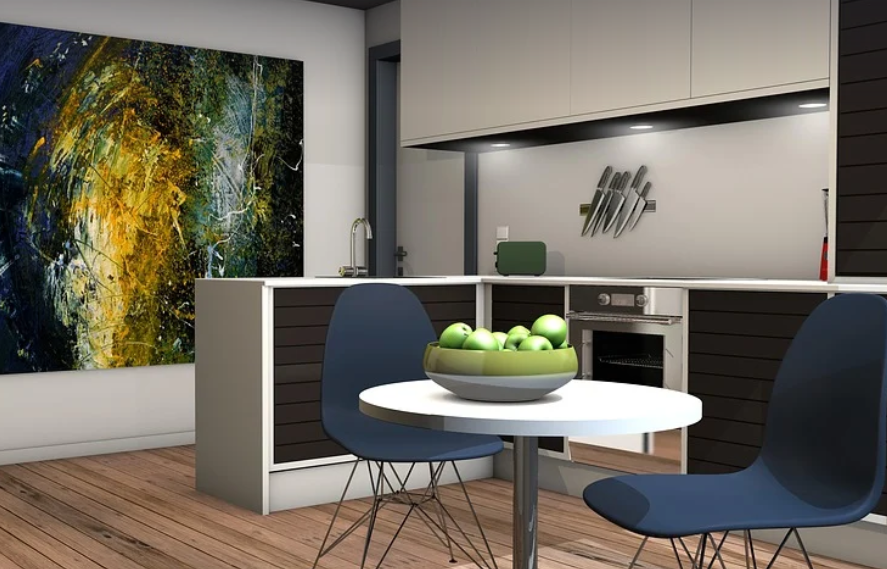
Dive Into Java Programming And Data Structures: A Beginner's Guide
What is this all about?
So, you’re thinking of venturing into the world of programming? Awesome! Welcome aboard. This guide will take you through the fundamentals of Java programming and data structures, laying a solid foundation for your coding journey.
Java, a popular and versatile programming language, offers immense possibilities in software development, ranging from simple apps to complex enterprise systems. And with these concepts, you’ll be equipped to build anything your imagination can dream up!
Data structures are the building blocks of our code; they help us organize, store, and manipulate data efficiently. Understanding them is key to writing more optimized and elegant programs.
Don’t worry if you don’t have any coding experience. This guide will be your friendly companion as we explore these concepts step-by-step. We’ll start with the basics of Java programming, then dive deep into data structures that are fundamental for programmers.
Why Dive into Java Programming?
Java is incredibly popular in the tech world because of its flexibility and power:
* **Cross-Platform Compatibility:** Java codes work on Windows, Mac, Linux, Android devices, and more. * **Object-Oriented Paradigm:** Java’s focus on objects makes it easy to understand and build complex programs by breaking them down into manageable units.
* **Strong Community & Resources:** There’s a huge community of Java developers ready to help you out, with vast online resources, forums, and tutorials available at your fingertips.
Java Programming Essentials: Your Roadmap
Let’s start with the essentials!
* **Hello World!:** The “Hello World!” program is a classic starting point for any programming journey. It teaches you how to write code and display output on the screen.
* **Variables, Data Types & Operators:** Think of these as containers that hold information. You’ll learn about different types of data (like numbers, text, true/false values) and how to use operators for simple calculations.
* **Control Flow: ** This is where you tell your program what to do in a sequence – “if this happens, then do that,” or “do this until that occurs” – all thanks to conditional statements like “if”, “else if,” and “else,” along with loops for repetition.
* **Functions:** We’ll learn how to break down complex tasks into smaller pieces using functions. They make your code more reusable and manageable. You can even pass arguments to them!
* **Arrays: Your Data Storage Heroes:** Arrays are like organized lists of items that can be accessed by their index. Imagine storing a shopping list or a set of exam scores; arrays make it easy to organize.
* **Classes and Objects: Building Blocks of Java** Class is the blueprint for an object, defining its properties (data) and behaviors (actions). Think of objects as specific instances created based on these blueprints.
* **More Advanced Topics:** As you progress, we’ll explore more advanced concepts like exception handling, file input/output, multithreading, and networking. You’ll start seeing how to connect with data sources like databases.
Data Structures: Building the Framework
Let’s talk about the heart of your programs – Data Structures!
Data structures are the way we organize and store data, making our code more efficient. Imagine you have a box full of toys. It would be messy to just throw everything in one big pile. Instead, you’d categorize them for better access: blocks with construction playsets, dolls, action figures, etc.
Data structures help us organize this information in a structured way:
* **Arrays:** A simple data structure where items are stored in a sequential order. Imagine your toy boxes – they have a specific order to them, right?
* **Linked Lists:** Think of a chain of linked objects; each object can point to the next one – like a list of friends you’d put on a line.
* **Stacks and Queues:** These structures play a role in tasks involving order, much like how we might arrange groceries or wait for our turn at the checkout counter.
* **Trees & Graphs:** Think of this as a family tree – each node represents a person (in your case, data), and branches connect them. This is great for representing hierarchical relationships in your programs.
Conclusion: Your Journey Begins
So, there you have it! The fundamentals of Java programming and data structures are just the tip of the iceberg. The real magic happens when you start applying these concepts to build your own unique projects, solve complex problems, and contribute to a vibrant tech community.
Don’t be afraid to jump in, explore, experiment, and most importantly, have fun!
Happy coding!